AeroGear Crypto Spec
by Bruno Oliveira
Good morning, today was created the following branch
https://github.com/aerogear/aerogear.org/tree/crypto-doc with our
discussions and also I included what Matthias sent to the mailing list.
This branch is a live copy of the document and not ready to be merged
until the whole team agree. So please feel free to send PRs, correcting
typos, add sections.
--
abstractj
10 years, 9 months
Cookbook for iOS
by Corinne Krych
Hello Guys
I like very Android Cookbook repo and I like to start the same idea for iOS. Putting together receipes in one common repo with different exemples sorted by folder. Adaopting the naming convention "HowTo…." for the file name that will match the documentation "How to … " is a good ay to easily find the information you need.
Talking about it on IRC we had a question though: how do we handle when in the exemple you need a server side code.
Would you deploy it on cloud server and available for client apps. Like in https://github.com/aerogear/aerogear-android-cookbook/blob/master/src/org...
Or would you package the server side exemple in the cookbook repo?
I rather put servide side in cookbook as we could also to demo this part too.
We have this need for example with the multipar file upload example:
https://github.com/cvasilak/MultiPartDemo
https://github.com/cvasilak/MultiPartIOSDemo
++
Corinne
10 years, 9 months
AeroGear Crypto API - Draft 0. Your brain is required
by Bruno Oliveira
Good morning all, just to start the discussion about the APIs and
encrypted storage I wrote this gist. Probably after some revisions I
hope to make it a specification.
Regarding the available scenarios, feel free to add or change the priority.
Gist: https://gist.github.com/abstractj/f1229ae075f8e6688c75
# AeroGear Crypto API
**Note**: This document is a working progress
# Authors
- Bruno Oliveira
- *put your pretty name here*
## Goals
- User friendly interface for non crypto experts
- Advanced developers can make use of the pure crypto provider
implementation.
## Supported Algorithms
- https://issues.jboss.org/browse/AGSEC-114
## Scenarios
**Note**: For all scenarios the authentication process was intentionally
ignored.
- A logged in user wants to store sensitive data on mobile
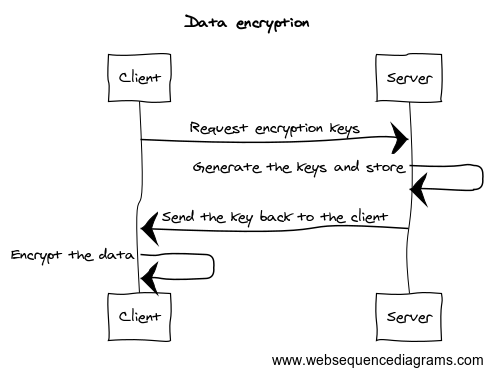
- The mobile device goes offline but the sensitive data must be safe
[Under development]
- Device was stolen and data must be destroyed
[Under development]
- The data must be backed up on the server, but passwords can't be exposed
[Under development]
- The application was installed into another device and the keys must be
revoked on the server
[Under development]
- User wants to configure for how long the keys will be considered valid
## JavaScript
### Dependencies
- [sjcl](http://crypto.stanford.edu/sjcl/) with wrappers for basic
functionalities like: encrypt, decrypt, password salting and key pair
generation.
### Implementation details
- The size of sjcl library is still a concern (28K)
- Crypto bits were built in a separate module so it may be
included/excluded in a custom build.
- The project will be developed under AeroGear.js repository
(https://github.com/aerogear/aerogear-js/pull/57)
### API (draft 0)
- Password based key derivation support (PBKDF2)
myEncryptedPassword = AeroGear.password("strong");
- Symmetric encryption support (GCM)
- Encryption:
var options = {
IV: superRandomInitializationVector,
AAD: "whateverAuthenticatedData",
key: generatedKey,
data: "My bonnie lies over the ocean"
};
var cipherText = AeroGear.encrypt( options );
- Decryption:
var options = {
IV: superRandomInitializationVector,
AAD: "whateverAuthenticatedData",
key: generatedKey,
data: cipherText
};
AeroGear.decrypt( options );
- Message authentication support (GMAC, HMAC)
[Under development]
**Note**: The implementations below are currently under discussion at
https://github.com/aerogear/aerogear-js/pull/62
- Hashing support (SHA-256, SHA-512)
digest = AeroGear.crypto.hash("some message");
- Asymmetric encryption support (ECC)
var hex = sjcl.codec.hex,
keyPair = new AeroGear.crypto.KeyPair(),
cipherText, plainText,
options = {
IV: superRandomInitializationVector,
AAD: "whateverAuthenticatedData",
key: keyPair.publicKey,
data: ""My bonnie lies over the ocean"
};
cipherText = AeroGear.crypto.encrypt( options );
options.key = keyPair.privateKey;
options.data = cipherText;
plainText = AeroGear.crypto.decrypt( options );
- Digital signatures support (ECDSA)
var validation,
options = {
keys: sjcl.ecc.ecdsa.generateKeys(192),
message: "My bonnie lies over the ocean"
};
options.signature = AeroGear.crypto.sign( options );
validation = AeroGear.crypto.verify( options );
## Android
### Dependencies
- [Spongy Castle](http://rtyley.github.io/spongycastle/) with wrappers
for basic functionalities like: encrypt, decrypt, password salting and
key pair generation.
### Implementation details
- The bouncycastle "provided" in Android doesn't have ECDH that's the
reason why Spongy Castle was chosen.
- aerogear-crypto-java will be the main repository to provide a crypto
API for Android and the Java server.
### API (draft 0)
**Note**: The implementations below are currently under discussion at
https://github.com/aerogear/aerogear-crypto-java/tree/refactoring
- Password based key derivation support (PBKDF2)
Pbkdf2 pbkdf2 = AeroGearCrypto.pbkdf2();
byte[] rawPassword = pbkdf2.encrypt(PASSWORD);
- Symmetric encryption support (GCM)
- Encryption:
CryptoBox cryptoBox = new CryptoBox(new
PrivateKey(SOME_SECRET_KEY));
final byte[] IV = new Random().randomBytes();
final byte[] message = "My bonnie lies over the
ocean".getBytes();
final byte[] ciphertext = cryptoBox.encrypt(IV, message);
- Decryption:
CryptoBox pandora = new CryptoBox(new
PrivateKey(SOME_SECRET_KEY));
final byte[] message = pandora.decrypt(IV, ciphertext);
- Message authentication support (GMAC, HMAC)
[Under development]
- Hashing support (SHA-256, SHA-512)
[Under development]
- Asymmetric encryption support (ECC)
KeyPair keyPair = new KeyPair();
KeyPair keyPairPandora = new KeyPair();
CryptoBox cryptoBox = new CryptoBox(keyPair.getPrivateKey(),
keyPairPandora.getPublicKey());
final byte[] IV = new Random().randomBytes();
final byte[] message = "My bonnie lies over the ocean".getBytes();
final byte[] ciphertext = cryptoBox.encrypt(IV, message);
CryptoBox pandora = new
CryptoBox(keyPairPandora.getPrivateKey(), keyPair.getPublicKey());
final byte[] message = pandora.decrypt(IV, ciphertext);
- Digital signatures support (ECDSA)
[Under development]
## iOS
### Dependencies
[TBD] - http://oksoclap.com/p/iOS_Meeting_(Security)
- [Common
Crypto](https://developer.apple.com/library/mac/documentation/security/co...
### Implementation details
[TBD]
### API (draft 0)
- Password based key derivation support (PBKDF2)
[Under development]
- Symmetric encryption support (GCM)
[Under development]
- Message authentication support (GMAC, HMAC)
[Under development]
- Hashing support (SHA-256, SHA-512)
[Under development]
- Asymmetric encryption support (ECC)
[Under development]
- Digital signatures support (ECDSA)
[Under development]
--
abstractj
10 years, 9 months
cordova plugins landed
by Erik Jan de Wit
Hi
The cordova plugins have landed under the aerogear base project:
The OTP cordova plugin with integrated barcode scanner aerogear-otp-cordova
The specialized push plugin for easy integration with Unified Push Server aerogear-pushplugin-cordova
Demo project of the aerodoc-web project wrapped in cordova together with the standard PushPlugin for the push messages aerogear-aerodoc-cordova
This is a step in the right direction for our efforts to support cordova developers more, but we need more and we don't need to support only the current modules we can create other handy plugins that are not there now. For example:
Crypto native storage
GeoTools Plugin (GeoFencing Maps)
Google Wallet Plugin (does iOS have something like that?)
Ads Plugin (one that combines iOS and Android)
WP8 support for all existing plugins
So please if you have some ideas about what else would be good to develop I would like to hear about it.
One issue still remain although these plugins contain tests and these tests are integration test and need to run on an emulator or on a actual device, do any of you have ideas how we can get that configured on travis. Or if you have other ideas how to test these plugins would be more then welcome.
Cheers, Erik Jan
10 years, 9 months
AeroGear Controller Demo - JAXRS
by Bruno Oliveira
There are several approaches, I think simple is good. So if is necessary
to create a class SampleUser, SimpleUser or whatever, go for it.
> Sebastien Blanc <mailto:scm.blanc@gmail.com>
> October 10, 2013 9:34 AM
> I was looking at the signature of the login
> method https://github.com/aerogear/aerogear-controller-demo/blob/jaxrs/src/main/...
>
> publicUserlogin(finalUseruser,Stringpassword)
>
> Currently it accepts an (PL) user and password String which does not
> fit very well with both JAX-RS and on how our Auth Client modules
> sends the data (JSON).
>
> Our AerogearUser firend is dead but for this repo shouldn't we have
> some kind of same class (just for internal use), just to hold the
> loginName and Password to make the flow easier ?
>
> Not sure what is the best approach here ...
>
> Seb
>
--
abstractj
10 years, 9 months
AG integration tests fail to get code coverage report
by Stefan Miklosovic
Hi,
I am getting error when generating code coverage report from aerogear-android-integration-tests.
After mvn clean install -Pemma all seems to be good but the actual genration fails with this:
http://pastebin.com/raw.php?i=u847VzC1
Manual generation of that report seems to be errorneous as well and it produces the same error ...
java -cp emma.jar emma report -r html -in coverage.em,coverage.ec
(pretty obvious since maven plugin for it does just the same thing).
1) Is here any hint how to get that generated?
2) Should not we abandon whole emma code coverage thing and stick to
something like jacoco? It is even possible to use jacoco for integration-tests repository?
3) Are there some other tools you would like to see be used in order to escape emma?
I noticed there is the dependency on emma4it-maven-plugin which has to be cloned from repository which was forked from the original one and where some changes were made. That forked repository was deleted some time ago so it does not exist anymore so I had to stick with the original one which can be the source of that error. Is there any way how we can rely on non-snapshot repos which do not get deleted randomly?
Stefan Miklosovic
Red Hat Brno
irc: smikloso
10 years, 9 months
Replacement of Aerogear-controller-demo Repo
by Sebastien Blanc
Hi,
Following the discussion we had yesterday during the team meeting about the
aerogear-controller-demo repository I've created this jira
https://issues.jboss.org/browse/AEROGEAR-1327
Maybe we could also use this thread to sum up what we need to expose as
rest endpoints in the new repo :
* auth : to test login / logout / enroll
* simple CRUD endpoints : to test paging (and implicitly the pipes)
* a OTP related thing endpoint
Did I miss something ?
Let's also start a poll on the repo name :
* aerogear-backend-demo
* aerogear-backend-support
ideas ?
Seb
10 years, 9 months
proper way to use AeroGear.Pipeline with parameter fed REST endpoint
by rnaszcyn
Hi all. I have started to use Aerogear for my mobile connectivity with pretty
good luck. One question I have is what is the best way to connect using
Aerogear to REST endpoints that accept a parameter. For example, I have the
following working snippet in a Javascript file I used for a mobile
application.
// TODO: change the pipeline name to use the school id passed to the page
var schoolMenuPipe = AeroGear.Pipeline([ {
name : "schoolmenu",
recordId : "1",
settings : {
baseURL : "/harpsterserver/rest/p1/",
endpoint : "schoolmenu/1" // The school ID is temporarily hard coded!!
}
} ]).pipes.schoolmenu;
In order to get the parameter into the Pipeline call (in this case a school
ID) I have to include the endpoint in the settings object so the default
pipeline name won't be used for the endpoint.
The REST endpoint is running on EAP and is hard coded with a return value
now for prototype purposes.
@GET
@Path("/school/{id}")
@Produces("application/json")
public School getSchool(@PathParam("id") Long id){
// TODO: add code that will search for a specific school
return new School(2, "Marist High School");
}
Everything is working fine right now but leaves me wondering if I have a
good solution pattern. I am new to Aerogear so perhaps I am missed some
documentation or code example.
--
View this message in context: http://aerogear-dev.1069024.n5.nabble.com/proper-way-to-use-AeroGear-Pipe...
Sent from the aerogear-dev mailing list archive at Nabble.com.
10 years, 9 months